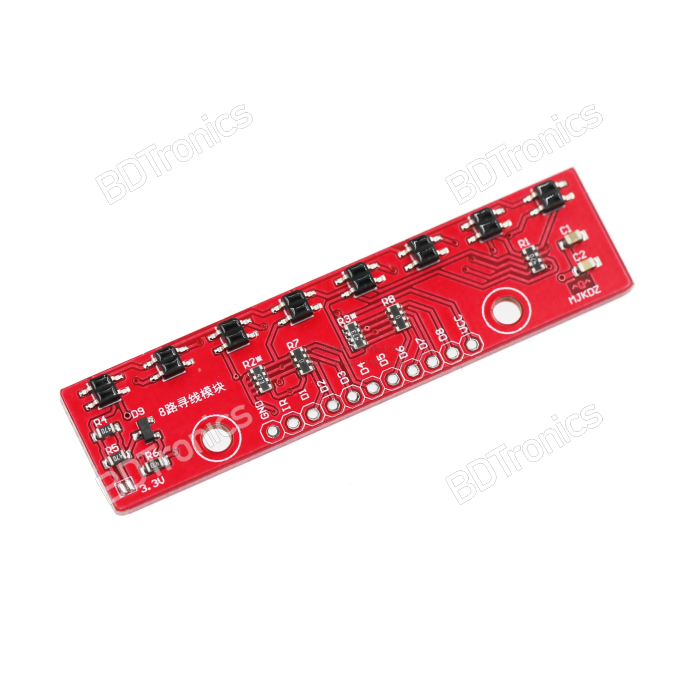
8 Channel IR Tracking Sensor Module Infrared Line Tracker for Arduino Smart Car Line Follower Robot
In stock
Order before 12.00PM
Product Details / 8 Channel IR Tracking Sensor Module Infrared Line Tracker for Arduino Smart Car Line Follower Robot
Specifications:
- Model: Tracking Sensor Module
- Working voltage:5V
- Channel: 8-channel
- PCB Color: Red
Application:
Package Included:
Request Stock
General Questions
What is the price of the 8 Channel IR Tracking Sensor Module Infrared Line Tracker for Arduino Smart Car Line Follower Robot in Bangladesh?
The latest price of 8 Channel IR Tracking Sensor Module Infrared Line Tracker for Arduino Smart Car Line Follower Robot in Bangladesh is BDT 390.00 . You can buy the 8 Channel IR Tracking Sensor Module Infrared Line Tracker for Arduino Smart Car Line Follower Robot at the best price on BDTronics.com or contact us via phone.
Where to buy 8 Channel IR Tracking Sensor Module Infrared Line Tracker for Arduino Smart Car Line Follower Robot in Bangladesh?
You can buy 8 Channel IR Tracking Sensor Module Infrared Line Tracker for Arduino Smart Car Line Follower Robot online by ordering on our website or directly collect by visiting our store in person. We provide home delivery service all over BD with cash on delivery and online payment method. It usually takes 1-2 days to deliver inside Dhaka and 2-3 days outside Dhaka.